iframe to HTML converter
Table of Contents
What is an Iframe?
An iframe is an HTML element that embeds another webpage within the current webpage. It is commonly used to display external content such as videos, maps, forms, and advertisements without redirecting the user.
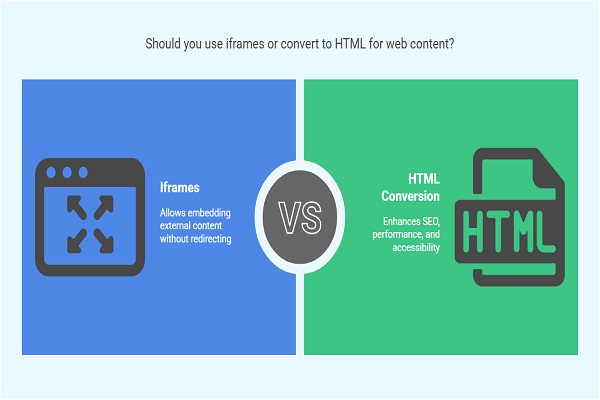
Basic Iframe Syntax
<iframe src="https://example.com" width="600" height="400" title="Example iframe"></iframe>
The src attribute defines the URL of the content to embed, while width and height specify the size of the iframe. The title attribute improves accessibility.
Why Convert Iframe to HTML?
Iframe content loads separately from the main page, which can cause performance and SEO issues. Converting an iframe to HTML helps resolve these problems.
Benefits of Converting Iframe to HTML
- SEO Optimization: Search engines may not fully index iframe content, reducing visibility in search results.
- Better Page Performance: Removing iframes reduces HTTP requests, improving page speed.
- Improved Accessibility: Direct HTML content ensures compatibility with screen readers and assistive technologies.
- Increased Customization: You gain complete control over styling and scripting.
Methods to Convert Iframe to HTML
There are multiple ways to extract and embed iframe content directly into a webpage.
1. Manual Extraction
If the content inside an iframe is accessible, copy and paste the HTML into your page.
<div>
<h2>Embedded Content</h2>
<p>This is the content that was inside the iframe.</p>
</div>
Ensure you include necessary CSS and JavaScript files.
2. Server-Side Fetching
A server-side script can retrieve and display the iframe content dynamically.
Example Using PHP:
<?php
$html = file_get_contents('https://example.com/page-to-embed');
echo $html;
?>
This fetches the source page’s HTML and embeds it directly.
3. JavaScript-Based Content Extraction
JavaScript can extract and embed iframe content on the client side.
Example Using JavaScript:
<iframe id="myIframe" src="https://example.com" style="display: none;"></iframe>
<div id="content"></div>
<script>
document.getElementById("myIframe").onload = function() {
var iframe = document.getElementById("myIframe").contentWindow.document.body.innerHTML;
document.getElementById("content").innerHTML = iframe;
};
</script>
This script extracts iframe content and displays it inside a div.
4. Using API or JSON Data
If the iframe loads content from an API, fetch the data directly.
Example Using Fetch API:
<script>
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
document.getElementById("content").innerHTML = data.html;
});
</script>
<div id="content"></div>
This method ensures efficient data retrieval without embedding full pages.
Considerations When Converting Iframe to HTML
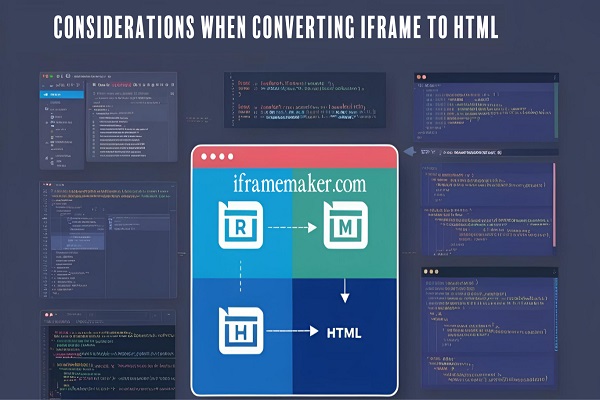
1. Legal and Ethical Aspects
- Ensure you have permission to use and modify content from an external source.
- Some websites restrict access to their content, preventing direct embedding.
2. Handling Dynamic Content
- If the iframe updates frequently, consider automating the extraction process using AJAX or server-side scripts.
3. Maintaining Design and Functionality
- Ensure styles and scripts from the original source are included.
- Test responsiveness on different devices.
Alternative Solutions
If you cannot convert an iframe to HTML, consider alternative embedding methods:
1. Embed with Custom Styling
Modify iframe properties to blend with your design.
<iframe src="https://example.com" style="border: none; width: 100%; height: 500px;"></iframe>
2. Use API Instead of Iframe
Many services provide APIs that allow direct content integration without iframes.
3. Load External Content Dynamically
Use AJAX to fetch and display content without an iframe.
<script>
document.getElementById("loadContent").addEventListener("click", function() {
fetch('https://example.com/page-to-embed')
.then(response => response.text())
.then(data => document.getElementById("content").innerHTML = data);
});
</script>
<button id="loadContent">Load Content</button>
<div id="content"></div>
Conclusion
Converting an iframe to HTML improves SEO, page speed, and accessibility. Use manual extraction, server-side fetching, JavaScript, or API-based methods for seamless content integration. Choose a method that aligns with your website’s structure and performance goals.